Paid Feature
This is a paid feature.
For self hosted users, Sign up to get a license key and follow the instructions sent to you by email. Creation of tenants is free on the dev license key.
This feature is already enabled for managed service users. Creation of additional tenant is free on the provided development environment.
Creating a new tenant
- Dashboard
- NodeJS
- GoLang
- Python
- cURL
Important
import Multitenancy from "supertokens-node/recipe/multitenancy";
async function createNewTenant() {
let resp = await Multitenancy.createOrUpdateTenant("customer1", {
firstFactors: ["emailpassword", "thirdparty", "otp-email", "otp-phone", "link-phone", "link-email"]
});
if (resp.createdNew) {
// Tenant created successfully
} else {
// Existing tenant's config was modified.
}
}
- In the above, we are creating a new tenant with the id
"customer1"
. We are also enabling the email password, third party and passwordless login methods for this tenant. You can also disable any of these by not including them in thefirstFactors
input. IffirstFactors
is not specified, by default, none of the login methods are enabled. - You can also set
firstFactors
tonull
in which case SDK will be able to use any of the login methods.
The built-in Factor IDs that can be used for firstFactors
are:
- Email password auth:
emailpassword
- Social login / enterprise SSO auth:
thirdparty
- Passwordless:
- With email OTP:
otp-email
- With SMS OTP:
otp-phone
- With email magic link:
link-email
- With SMS magic link:
link-phone
- With email OTP:
import (
"github.com/supertokens/supertokens-golang/recipe/multitenancy"
"github.com/supertokens/supertokens-golang/recipe/multitenancy/multitenancymodels"
)
func main() {
tenantId := "customer1"
emailPasswordEnabled := true
thirdPartyEnabled := true
passwordlessEnabled := true
resp, err := multitenancy.CreateOrUpdateTenant(tenantId, multitenancymodels.TenantConfig{
EmailPasswordEnabled: &emailPasswordEnabled,
ThirdPartyEnabled: &thirdPartyEnabled,
PasswordlessEnabled: &passwordlessEnabled,
})
if err != nil {
// handle error
}
if resp.OK.CreatedNew {
// new tenant was created
} else {
// existing tenant's config was modified.
}
}
- In the above, we are creating a new tenant with the id
"customer1"
. We are also enabling the email password, third party and passwordless login for this tenant. You can also disable any of these by setting the corresponding field tofalse
(which is also the default setting).
- Asyncio
- Syncio
from supertokens_python.recipe.multitenancy.asyncio import create_or_update_tenant
from supertokens_python.recipe.multitenancy.interfaces import TenantConfig
async def some_func():
response = await create_or_update_tenant("customer1", TenantConfig(
email_password_enabled=True,
third_party_enabled=True,
passwordless_enabled=True,
))
if response.status != "OK":
print("Handle error")
elif response.created_new:
print("New tenant was created")
else:
print("Existing tenant's config was updated")
from supertokens_python.recipe.multitenancy.syncio import create_or_update_tenant
from supertokens_python.recipe.multitenancy.interfaces import TenantConfig
response = create_or_update_tenant("customer1", TenantConfig(
email_password_enabled=True,
third_party_enabled=True,
passwordless_enabled=True,
))
if response.status != "OK":
print("Handle error")
elif response.created_new:
print("New tenant was created")
else:
print("Existing tenant's config was updated")
- In the above, we are creating a new tenant with the id
"customer1"
. We are also enabling the email password, third party and passwordless login for this tenant. You can also disable any of these by setting the corresponding field tofalse
(which is also the default setting).
- Single app setup
- Multi app setup
- Core version >= 9.1.0
- Core version <= 9.0.2
curl --location --request PUT '/recipe/multitenancy/tenant/v2' \
--header 'api-key: ' \
--header 'Content-Type: application/json' \
--data-raw '{
"tenantId": "customer1",
"firstFactors": ["emailpassword", "thirdparty", "otp-email", "otp-phone", "link-email", "link-phone"]
}'
- We specify the appId for which we want to create a new tenant in the URL. If you are using the default (
"public"
) app, you can omit the/appid-<APP_ID>
part of the URL. - In the above, we are creating a new tenant with the id
"customer1"
. We are also enabling the email password, third party and passwordless login methods for this tenant. You can also disable any of these by not including them in thefirstFactors
array. IffirstFactors
is not specified, by default, none of the login methods will be enabled. - You can also set
firstFactors
tonull
in which case SDK will be able to use any of the login methods.
The built-in Factor IDs that can be used for firstFactors
are:
- Email password auth:
emailpassword
- Social login / enterprise SSO auth:
thirdparty
- Passwordless:
- With email OTP:
otp-email
- With SMS OTP:
otp-phone
- With email magic link:
link-email
- With SMS magic link:
link-phone
- With email OTP:
curl --location --request PUT '/recipe/multitenancy/tenant' \
--header 'api-key: ' \
--header 'Content-Type: application/json' \
--data-raw '{
"tenantId": "customer1",
"emailPasswordEnabled": true,
"thirdPartyEnabled": true,
"passwordlessEnabled": true
}'
- We specify the appId for which we want to create a new tenant in the URL. If you are using the default (
"public"
) app, you can omit the/appid-<APP_ID>
part of the URL. - In the above, we are creating a new tenant with the id
"customer1"
. We are also enabling the email password, third party and passwordless login for this tenant. You can also disable any of these by setting the corresponding field tofalse
(which is also the default setting).
- Core version >= 9.1.0
- Core version <= 9.0.2
curl --location --request PUT '/recipe/multitenancy/tenant/v2' \
--header 'api-key: ' \
--header 'Content-Type: application/json' \
--data-raw '{
"tenantId": "customer1",
"firstFactors": ["emailpassword", "thirdparty", "otp-email", "otp-phone", "link-email", "link-phone"]
}'
- We specify the appId for which we want to create a new tenant in the URL. If you are using the default (
"public"
) app, you can omit the/appid-<APP_ID>
part of the URL. - In the above, we are creating a new tenant with the id
"customer1"
. We are also enabling the email password, third party and passwordless login methods for this tenant. You can also disable any of these by not including them in thefirstFactors
array. IffirstFactors
is not specified, by default, none of the login methods will be enabled. - You can also set
firstFactors
tonull
in which case SDK will be able to use any of the login methods.
The built-in Factor IDs that can be used for firstFactors
are:
- Email password auth:
emailpassword
- Social login / enterprise SSO auth:
thirdparty
- Passwordless:
- With email OTP:
otp-email
- With SMS OTP:
otp-phone
- With email magic link:
link-email
- With SMS magic link:
link-phone
- With email OTP:
curl --location --request PUT '/recipe/multitenancy/tenant' \
--header 'api-key: ' \
--header 'Content-Type: application/json' \
--data-raw '{
"tenantId": "customer1",
"emailPasswordEnabled": true,
"thirdPartyEnabled": true,
"passwordlessEnabled": true
}'
- We specify the appId for which we want to create a new tenant in the URL. If you are using the default (
"public"
) app, you can omit the/appid-<APP_ID>
part of the URL. - In the above, we are creating a new tenant with the id
"customer1"
. We are also enabling the email password, third party and passwordless login for this tenant. You can also disable any of these by setting the corresponding field tofalse
(which is also the default setting).
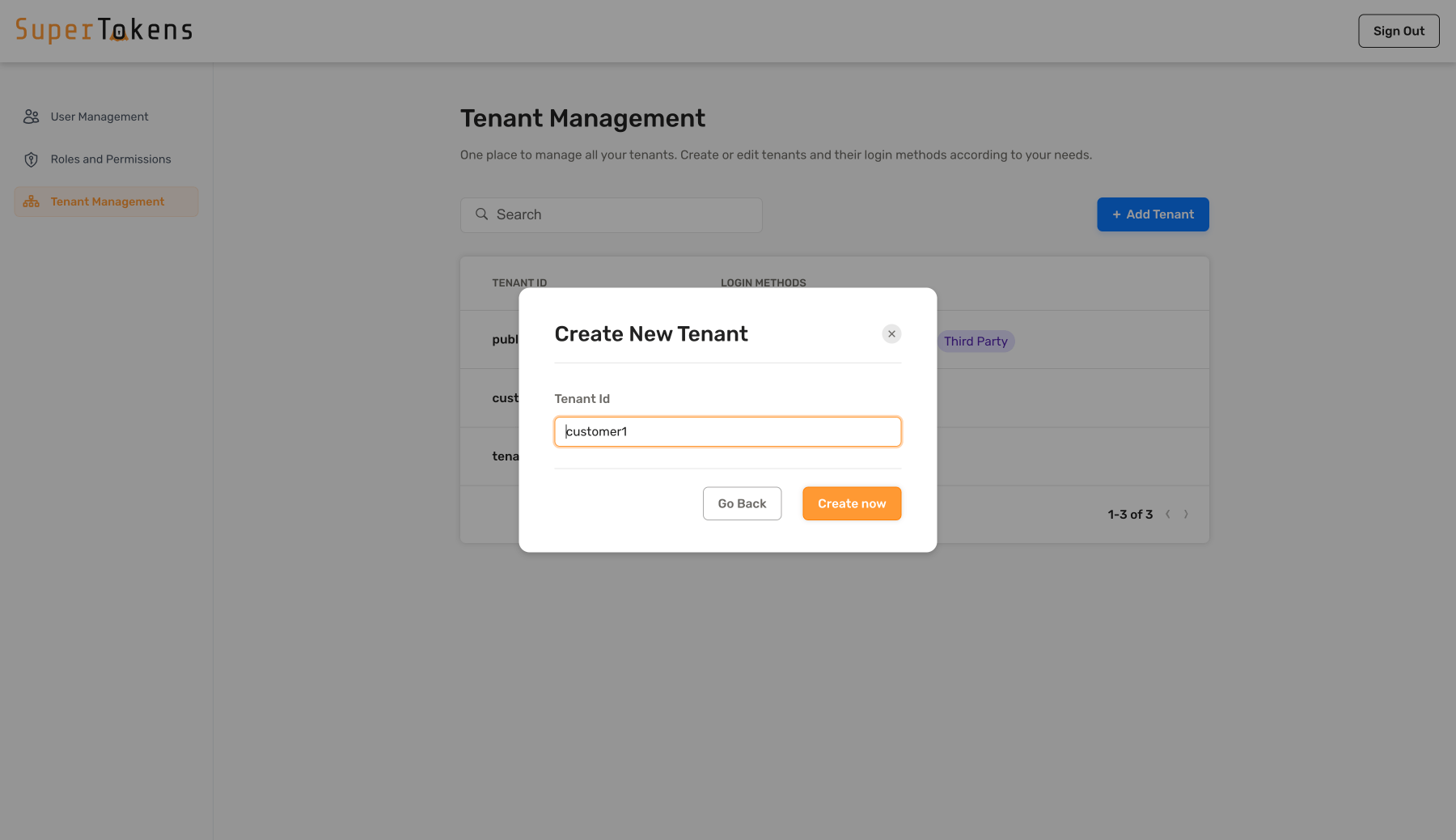
Create a new tenant by clicking on the Add Tenant button and specify the tenant ID.
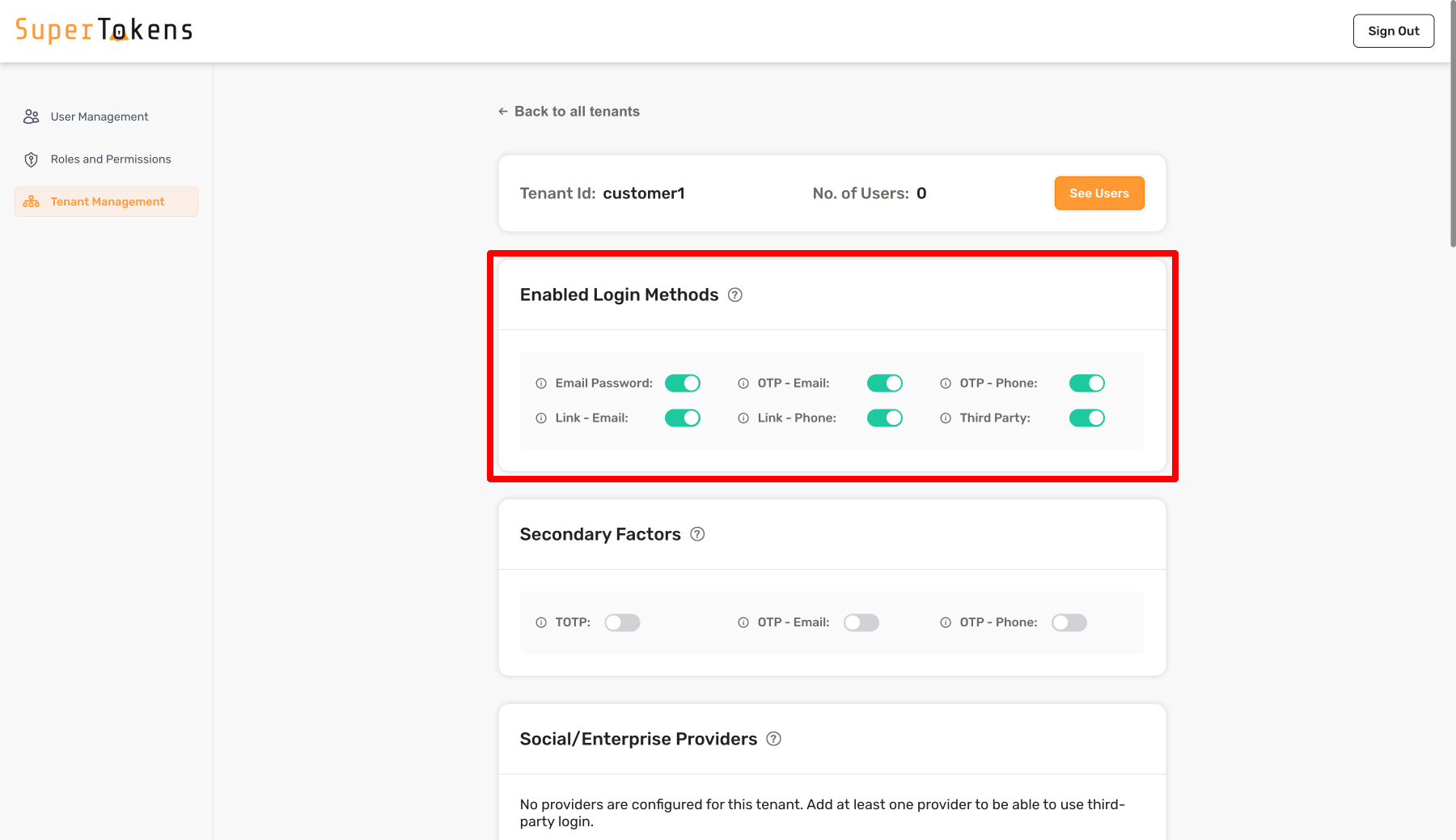
Once the tenant is created, turn on the Login Methods as required for the tenant. In the above example, all the Login Methods are turned on.
#
Next stepsCheckout the "Setting up login for tenants" page for next steps in integrating multi tenancy.